Whenever we choose the Export to Excel option from our Application, a dialog box pops us with the option to Open or to Save, as shown below:
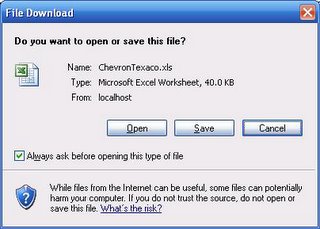
By chance if the user checks off the option "Always ask before opening this type of file", from next time the user will not be able to see the dialog box. Instead, the excel file opens up in the same window.
To set back this option, the following steps can be followed:
1. Go to Windows Explorer.
2. On the Tools menu, click Folder Options, and then click on the File Types tab.
3. From the Registered file types list box, select the XLS extension, and then click Advanced.
4. In the Edit File Type dialog box, set the Confirm open after download to selected.
5. Make sure the Browse in same window option is not selected, and then click OK.
The above steps will make sure that we get the dialog box as shown above. However, since this is an option set at the client computer, these steps cannot be mandated to be followed in every computer that browses the application.
So, from the code level, we must make sure that the excel file is opened in a separate window. One possible option for this is to Save the file to the web server, and then open the file in a separate window.
The code for this is given below:
{
try
{
string strFileName = String.Empty, strFilePath= String.Empty;
strFilePath = Server.MapPath(@"../Excel/") + "ExcelFileName" + ".xls";
if (File.Exists(strFilePath))
{
File.Delete(strFilePath);
}
System.IO.StringWriter oStringWriter =new StringWriter();
System.Web.UI.HtmlTextWriter oHtmlTextWriter = new HtmlTextWriter(oStringWriter);
StreamWriter objStreamWriter;
string strStyle =@"
";
objStreamWriter = File.AppendText(strFilePath);
dgExport.RenderControl(oHtmlTextWriter);
objStreamWriter.WriteLine(strStyle);
objStreamWriter.WriteLine(oStringWriter.ToString());
objStreamWriter.Close();
string strScript = "<script language=JavaScript>window.open('../Excel/" + "ExcelFileName" +
".xls','dn','width=1,height=1,toolbar=no,top=300,left=400,right=1,
scrollbars=no,locaton=1,resizable=1');</script>";
if(!Page.IsStartupScriptRegistered("clientScript"))
{
Page.RegisterStartupScript("clientScript", strScript);
}
}
catch(Exception)
{
//Handle Exception
}
}
In the above method, the file is saved to the Web Server inside the folder "Excel". Of course, this folder must have write permissions for the user. But it will definitely ensure that the excel file is opened in a new window in the client computer.
Cheers.
7 comments:
Hi Lakshmi,
Thanks for the code.
I used the code in my application.
When I retrive the .xls page, it is retriving the old page which is stored in the temporary internet files, even though the new .xls page is beging stored in the folder.
If i go to tools/ internet options/temperory internet files
and delete then, then it is retrevign the new version of the page.
Please let me know how to deal with this problem.
Its really urgent.
Reply to me at i.netdeveloper@gmail.com
Thanks
After data is saved in file which in Server path, I am unable to delete the file which is in server path. Code is not executed after window is displayed for save.
Thanks,
Appan.
This works abs fine but my requirement is to show the Data in the instance of the excel instead of IE. I am using IE 6.0 and seems nothing working for it.
Any help will be appreciated
Thanks
Gourav
gourav1980@gmail.com
You are the man! This helped me out big time :)
cooollllllll...Thanks a lottt dude...u saved me big time....
If you have this situation,then I recommend to use this application-Microsoft Excel the file error: there is a possibility that data is lost.,which in my view one of the best in this sphere,it has free status,also software is used to process important information, such as graphics, diagrams, commercial and statistical data,recover *.xlsx, *.xlsm, *.xltm, *.xltx or *.xlam formats,tool will help you if you have to repair Excel file error message: Excel error the file is not in a recognizable format,allows choosing a file, that shown an error like: Excel error message this file is not in a recognizable format, then, you should fix Excel file for errors and proceed with its analysis,attempts to recover your document with this message: Excel error and this file is not in a recognizable format and shows a preview window.
Any idea with the missing css file issue? I could not find it any where.
Thanks,
Sharmin
Post a Comment